1. Problem statement
Given an array A of integers, reverse this array A in linear running time using constant memory.
2. Solution
The steps to reverse an array can be shown with the help of following figure:
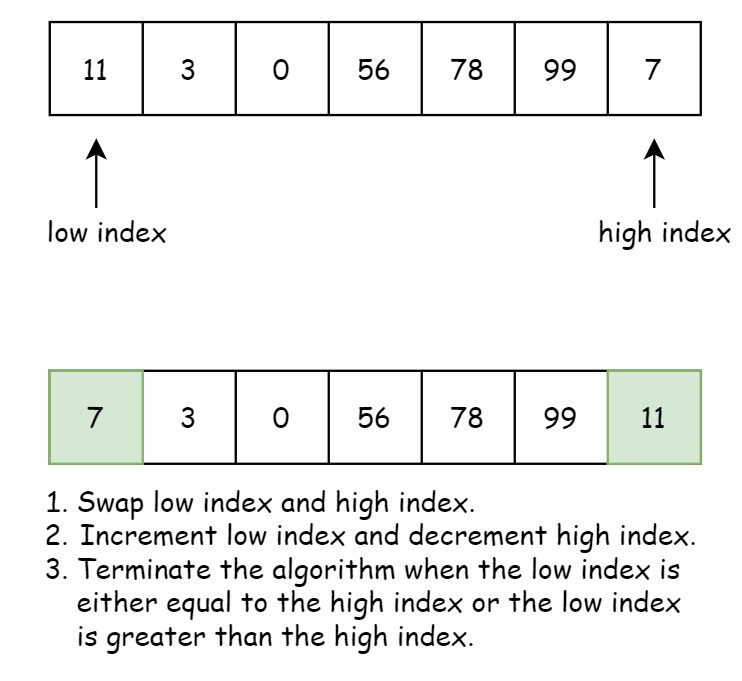
3. Program
Following is the program to reverse the array.
import java.util.Arrays; public class ReverseArray { public int[] reverse(int[] nums) { // initialize low and high index int lowIndex = 0; int highIndex = nums.length - 1; // while lowIndex is less than high index while (lowIndex < highIndex) { // swap low index and high index swap(nums, lowIndex, highIndex); //increment low index and decrement high index lowIndex++; highIndex--; } return nums; } private void swap(int[] nums, int index1, int index2) { int temp = nums[index1]; nums[index1] = nums[index2]; nums[index2] = temp; } public static void main(String[] args){ int[] nums = {1,2,3,4,5}; ReverseArray reverseArray = new ReverseArray(); int[] reversedArray = reverseArray.reverse(nums); System.out.println(Arrays.toString(reversedArray)); } }
Output:
[5, 4, 3, 2, 1]