1. Introduction
The main purpose of pipes is transformation. Pipes are used for transformation of strings, dates, currency amounts and other data. Pipe’s main purpose is only for display purpose. Pipes are used as reusable functionality which are declared only once and reused in the application.
Original value ——> Pipe ——> Transformed value
You can think of Pipes as reusable functions to transform values. A common place of using pipes is template expressions. In Angular, Pipe is a class which is preceded by the @Pipe{}
decorator. This class defines a function to transform input values to output values.
Following is a typical example of pipe:
<p>Start date: {{ startDate | date }}</p>
Here startDate
, is a date in the component TypeScript file.
birthday = new Date(1987, 4, 27);
2. Angular built-in pipes
Following are few built-in pipes:
- UpperCasePipe: Pipe to transform text to all upper case.
- LowerCasePipe: Pipe to transform text to all lower case.
- DatePipe: Pipe to format a date value according to locale rules.
- CurrencyPipe: Pipe to transform a number to a currency string, formatted according to locale rules.
- DecimalPipe: Pipe to transform a number into a string with a decimal point, formatted according to locale rules.
- PercentPipe: Pipe to transform a number to a percentage string, formatted according to locale rules.
3. Using a pipe in a template
To use a pipe in template expression, use |
and the name of the pipe like the following:
<p>Start date: {{ startDate | date }}</p>
src/app/app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], }) export class AppComponent { title = 'Pipe example'; lowercaseValue = 'hello world'; uppercaseValue = 'HELLO WORLD'; startDate = new Date(1987, 4, 27); currencyNumber: number = 0.359; percentageNumber: number = 0.459; }
src/app/app.component.html
<h1> {{ title }} </h1> <ul> <li><b>Tranformed uppercase value:</b> {{ lowercaseValue | uppercase }}</li> <li><b>Tranformed lowercase value:</b> {{ uppercaseValue | lowercase }}</li> <li><b>Tranformed date:</b> {{ startDate | date }}</li> <li><b>Tranformed currency:</b> {{ currencyNumber | currency }}</li> <li><b>Tranformed percent:</b> {{ percentageNumber | percent }}</li> </ul>
Output in browser
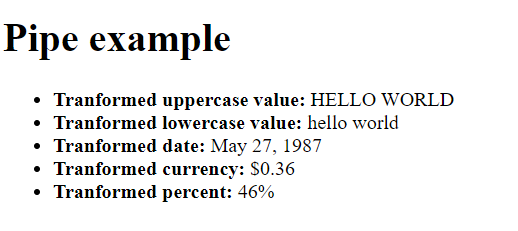
4. Using parameters with pipes
You can provide optional parameters to the pipe. Optional parameters are provided to further change the transformed value. To provide the optional parameter value, follow the pipe name with a colon (:
) and the parameter value. For example:
<p>Start date: {{ startDate | date: "medium" }}</p>
Transformed value looks like: May 27, 1987, 12:00:00 AM
If the pipe accepts multiple parameters, you can provide values separated with colons (:
). For example:
<b>Tranformed currency:</b> {{ currencyNumber | currency: "CAD":"code" }}
Transformed value looks like: CAD0.36
5. Chaining pipes
You can chain pipes as well so that the output of one pipe becomes the input to the next. For example:
<li><b>Tranformed date in uppercase:</b> {{ startDate | date | uppercase }}</li>
Transformed value looks like: MAY 27, 1987
6. Pipes and precedence
he pipe operator has a higher precedence than the ternary operator (?:
). So a ? b : c | pipe
is same as a ? b : (c | pipe)
. If you want to use pipe with the result of the pipe, then use the parentheses, (a ? b : c) | pipe
.
7. Conclusion
In this tutorial, we discussed Pipe in Angular. We discussed how to used a pipe, use optional parameters with pipe as well as a chaining of Pipe. This is not a complete tutorial. There are other things which you should read about like creating a custom Pipe. We’ll discuss all these topics in upcoming tutorials.