1. Introduction
Pipes are used for transformation of values. Angular provides several built-in pipes but sometimes the built-in pipes are not able to fulfill our specific requirement. Angular allows you to create your own custom pipe.
Pipe is a class decorated with @Pipe
decorator and a function to transform the value. This decorator @Pipe
marks a class as pipe. A pipe class must implement the PipeTransform
interface. A pipe class must implement the PipeTransform
interface. This interface has one method transform
which is invoked by Angular to transform value.
interface PipeTransform { transform(value: any, ...args: any[]): any }
2. Custom pipe example – pipe to decorate string
In this tutorial, we’ll create a custom pipe to prefix and suffix a string with Asterisk (*). We’ll provide how many * we want to suffix and prefix the string.
Step 1: Create an application
Create a new application pipe-example
by executing following command:
ng new pipe-example
Step 2: Add a pipe DecorateStringPipe by executing following command:
ng generate pipe DecorateString
This will generate following pipe:
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'decorateString', }) export class DecorateStringPipe implements PipeTransform { transform(value: string, count: number): string { let repeatStr: string = '*'.repeat(count); return repeatStr + value + repeatStr; } }
tranform
method accepts two arguments, value
is the value which will be transformed, count
is the optional argument. In order to use pipe in the template, add pipe in the declarations
array of the NgModule
. However, ng generate pipe
command automatically registers pipe in the NgModule
.
Step 3: Use pipe in template
Update app.component.html
with the following code:
<p><b>Original value:</b> {{ title }}</p> <p><b>Transformed value:</b> {{ title | decorateString: 5 }}</p>
Step 3: Run the application
Run the application using command ng serve
. The output in the browser looks like the following:
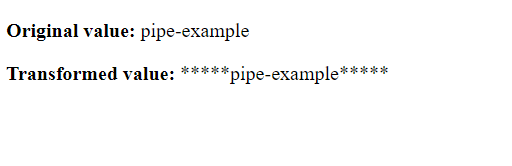
3. Conclusion
In this tutorial, we created a custom pipe. It is good to have knowledge of creating a custom pipe as pipes are usable and can be used in the entire application.
We hope this tutorial was informative.