1. Introduction
In this tutorial, we’ll discuss about Hibernate. This tutorial should be considered as an introduction to Hibernate framework. We’ll discuss the problems which Hibernate solves and its capabilities.
Hibernate is an Object/Relational Mapping solution for Java environments. This Object/Relational Mapping refers to the technique of mapping data from an object model representation to a relational data model representation (and vice versa).
2. Architecture
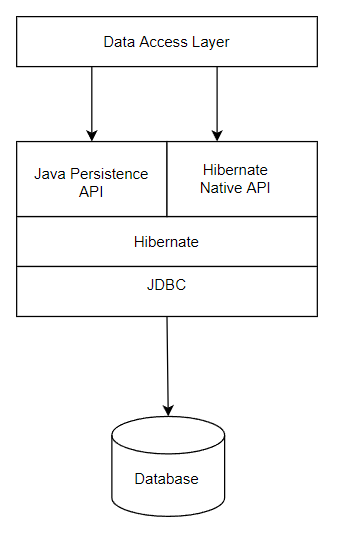
Hibernate sits between the Java application data access layer and the Relational Database and shown in the diagram. Java program uses Hibernate APIs to interact with database and perform operations like get, save, update and delete data.
3. What problems does Hibernate solve?
Following is a typical piece of JDBC code to connect database and get details:
import java.sql.*; public class FirstExample { static final String DB_URL = "dbc:mysql://localhost:3306/mydb"; static final String USER = "root"; static final String PASS = "admin"; static final String QUERY = "SELECT id, first, last, age FROM Employees"; public static void main(String[] args) { // Open a connection try(Connection conn = DriverManager.getConnection(DB_URL, USER, PASS); Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery(QUERY);) { // Extract data from result set while (rs.next()) { // Retrieve by column name System.out.print("ID: " + rs.getInt("id")); System.out.print(", Age: " + rs.getInt("age")); System.out.print(", First: " + rs.getString("first")); System.out.println(", Last: " + rs.getString("last")); } } catch (SQLException e) { e.printStackTrace(); } } }
If you observe there is code to retrieve data from the table. If required this data can be retrieved and set in an object. If Customer
is the class which needs to be populated from the table data, using Hibernate it can be done like this:
List<Customer> customerList = customerRepository.findAll();
Hibernate does all the translation from table data to the object graph.
4. What does Hibernate do?
Following are few capabilities of Hibernate:
- Hibernate takes care of mapping from Java classes to database tables and vice versa.
- Hibernate takes care of mapping Java data types to SQL data types.
- Hibernate provides data query and retrieval facilities.
- Hibernate enables you to write native SQL queries as well.
- Hibernate removes the need to write most of regular boiler-plate code to translate table data to the objects.
- Hibernate has the facility to manage table mappings which translate to object mappings like one-to-one, one-to-many and so on.
- Hibernate can automatically generate primary keys.
- Hibernate framework’s query language (HQL) is independent of database. So you can migrate to new database without re-writing queries.
- Hibernate supports caching of memory.