1. Introduction
You will often need to display different things on the screen based on some condition. In React, you can conditionally render JSX using JavaScript like syntax like if
statements, ?:
operators and &&
.
In React, control flow is handled by JavaScript.
2. Example
In this example, you can observe that a different JSX tree is returned based on the value of flag
.
Custom.js
function Custom({ name, flag }) { if (flag) { return ( <li> <b>{name}</b> </li> ); } else { return <li>{name}</li>; } } export default Custom;
App.js
import Custom from "./Custom"; function App() { return ( <ul> <Custom name="James" flag="true"></Custom> <Custom name="Roger" flag="false"></Custom> <Custom name="Ivanka" false="true"></Custom> </ul> ); } export default App;
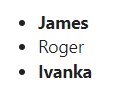
3. Conditionally returning nothing with null
Sometimes you may want to render nothing at all. In the same example mentioned earlier, if you do not want to render anything based on flag
, you can return null
.
Custom.js
function Custom({ name, flag }) { if (flag == true) { return ( <li> <b>{name}</b> </li> ); } else { return null; } } export default Custom;
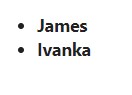
4. Conditional (ternary) operator (? : )
You can use ternary operator instead of using if
and else
in previous example.
function Custom({ name, flag }) { return ( <> {flag ? (<li><b>{name}</b></li>) : null} </> ); } export default Custom;
5. Logical AND operator (&&)
Another common shortcut you’ll encounter is the JavaScript logical AND (&&
) operator. The &&
operator is used in React when you want to render some JSX when the condition is true
, or render nothing otherwise.
You can use &&
operator to show some title, for example Mr.
Custom.js
function Custom({ name, flag }) { return ( <li> {flag && "Mr. "} {name} </li> ); } export default Custom;
App.js
import Custom from "./Custom"; function App() { return ( <ul> <Custom name="James" flag={true}></Custom> <Custom name="Roger" flag={true}></Custom> <Custom name="Ivanka" flag={false}></Custom> </ul> ); } export default App;
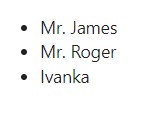
A JavaScript &&
expression returns the value of its right side (in our case, the title ‘Mr.’) if the left side (our condition, flag
) is true. But if the condition is false, the whole expression becomes false.
6. Don’t put numbers on the left side of &&
You may think that when 0 is provided to the left of &&
, React will not render anything. This is wrong assumption. For example,
count && <p>Some message here</p> // will render 0 if count is 0
Instead, you should use the following:
count > 0 && <p>Some message here</p>
7. Conditionally assigning JSX to a variable
In React, you can conditionally assign JSX to a variable. For example:
function custom({ name, flag }) { let itemContent = name; if (flag) { itemContent = "Mr. " + name; } return ( <li> {itemContent} </li> ); }
8. Conclusion
Conditional rendering is a fundamental concept in React that allows developers to create dynamic and interactive user interfaces. By mastering conditional rendering, you can make your applications more responsive to user interactions and changes in state or props.
In this tutorial, we explored various techniques for implementing conditional rendering in React. We covered:
- Using
if
Statements: A straightforward approach for simple conditions. - Ternary Operators: A concise way to handle simple conditional logic within JSX.
- Logical
&&
Operator: Useful for conditionally including elements based on a boolean value.
These methods enable you to control the display of components based on different conditions, improving the user experience by showing relevant content or hiding unnecessary details.
Remember that while conditional rendering is powerful, it is important to keep your components readable and maintainable. Overuse of conditional logic within a single component can make it difficult to manage. Instead, consider breaking down complex logic into smaller, reusable components.