1. Introduction
According to the documentation of Redux:
Redux is a JS library for predictable and maintainable global state management. Redux can be used with commonly used libraries like React, Angular, Vue.js. It helps manage the state of your application in a predictable way.
Redux is very small in size (2kB, including dependencies).
Redux Toolkit is the official recommended approach for writing Redux logic. It wraps around the Redux core, and contains packages and functions that we are essential for building a Redux app. Redux makes it easier to write Redux applications by simplifying writing code.
2. When should you use Redux?
There is no need to include Redux is every application. Redux solves specific problems. So whether to use Redux in your application or not completely depends on the type of application you are building.
Following are some cases when Redux is a good option:
- If the app is of medium or large size in terms of codebase and many developers working simultaneously on it.
- You have a significant amount of application state that is required in numerous parts of the app.
- The app state is updated frequently.
- The logic to update the state is complex.
- You need to keep a track of changes to the state over time.
3. When not to use Redux?
Redux is very powerful but need not to be used in every application. Here are some scenarios when you might not want to use Redux:
- Simple State Management: If your application requires minimal state management, Redux might be unnecessary. React’s built-in state management tools (
useState
anduseContext
) could be enough. - Small Applications: For small to medium-sized applications with limited complexity, the extra boilerplate and complexity that Redux introduces may not be warranted.
- Short-term Projects: For projects with a short lifecycle or for prototypes, the setup and learning curve of Redux can unnecessarily slow down development.
- Local State Management: If most of your state is local to individual components and not shared across many components, you might not need a global state management solution like Redux.
- Performance Concerns: In some instances, Redux can cause performance overhead because it requires components to re-render when the state changes. If performance is crucial and Redux isn’t providing optimal results, consider using alternative solutions.
- Learning Curve: If your team lacks experience with Redux, the learning curve can be quite steep. When state management needs don’t warrant this learning effort, it may be more practical to use simpler alternatives.
4. Core Concepts of Redux
- Store: The single source of truth that holds the state of your entire application.
- Actions: Plain JavaScript objects that describe what happened.
- Reducers: Functions that take the current state and an action, and return a new state.
- Dispatch: The method used to send actions to the store.
- Selectors: Functions that select a piece of the state.
5. Installation of Redux
Redux is available as a package on NPM.
npm install redux
Redux Toolkit is available as a package on NPM.
npm install @reduxjs/toolkit
6. Setup of Redux for this series of tutorials
We’ll have a separate setup for the learning Redux in this series of tutorials.
Following is the folder structure following with the code of the files. After setting up the folder structure and code. Navigate to the the ‘redux-starter’ folder and run npm install
. This will install all the dependencies on your machine.
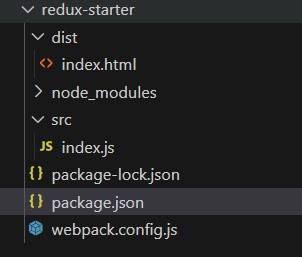
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Redux Project</title> </head> <body> <div id="app">Redux Starter Project</div> <script src="app.js"></script> </body> </html>
index.html
console.log("Redux Starter Project!!");
package.json
{ "name": "redux-starter", "version": "1.0.0", "description": "Redux Starter Project", "main": "index.js", "scripts": { "start": "webpack serve --config ./webpack.config.js" }, "keywords": [], "author": "", "license": "ISC", "devDependencies": { "webpack": "^5.89.0", "webpack-cli": "^4.10.0", "webpack-dev-server": "^4.15.1" }, "dependencies": { "redux": "^5.0.1" } }
webpack.config.js
const path = require("path"); module.exports = { entry: "./src/index.js", output: { filename: "app.js", path: path.resolve(__dirname, "dist"), }, devServer: { static: { directory: path.join(__dirname, "dist"), }, port: 3000, }, mode: "development", };
7. How React works?
React is used to maintain state of an application which is maintained in the Store. The store for a Social Media website may look like the following:
store = { user: [{...}], posts: [{...}], notifications: [{...}], friends: [{...}] }
However, it is completely up to us how we decide to design the Store.
An application has only one Redux store.
Reducer: Reducer is a pure function to used to update the store. Reducer takes the current state as an argument and returns the updated state. Reducer function is not supposed to make any side effects, so it should not make any HTTP calls or update DOM elements.
You may ask, how Reducer will know which task to perform. For this there are Actions. By Action a Reducer is informed about which task to perform. So there are 3 things to remember about Redux – Store, Reducer and Action.
Let us discuss how Redux works in an application.
Some action performed by the user triggers an event. This dispatches action to the Store. Store passes this action to the Store. Store then takes this action and decide which task to perform and updates the Store. So we can’t directly call reducers but only through Store. This creates a single entry point to the Store. This is helpful is auditing the actions performed on the Store.
8. Steps for implementing Redux
Following are the main steps to implement Redux:
- Designing the store
- List the actions. This defines what needs to be done.
- Create reducer function. This defines how to do.
- Create redux store.
9. Conclusion
In this short tutorial, we discussed Redux. We have also did the setup for the next series of tutorials for Redux.