1. Introduction
In this tutorial, we are going to discuss Spring Security. In this tutorial, we’ll discuss Spring Security in brief. Spring Security is a framework that provides authentication, authorization and protection against common attacks like CRSF.
Before we go ahead, let us discuss Authentication and Authorization.
2. What is Authentication?
Authentication is the process or action of proving or showing something to be true, genuine, or valid. Let us understand this with the help of an example. When you enter the premises of a company, you have to show your identity card. By seeing your identity card, the security guard can authenticate your identity.
Authentication verifies the identity of who is trying to access a resource or perform an action. A common way of authentication is using username and password. Spring Security provides built in support for authenticating users.
3. What is Authorization?
Authorization is to empower or permit someone to perform some action. Let us understand this with the help of an example. An employee of a company is not allowed to enter all departments of the office. For example, only employees who have responsibility to maintain salary account can be allowed to enter finance department. We can say that only finance department employees are authorized to enter finance department.
4. Features of Spring Security
Following are few features provided by Spring Security:
- Spring Security provides support for both Authentication and Authorization.
- Spring Security provides protection against attacks like CSRF, clickjacking, session fixation etc.
- Spring Security is available for servlet and web flux applications.
- Spring Security supports LDAP, OpenID authentication.
- Spring Security supports Single Sign-On) implementation.
- Spring Security supports “Remember-Me” feature.
5. Spring Security’s Architecture
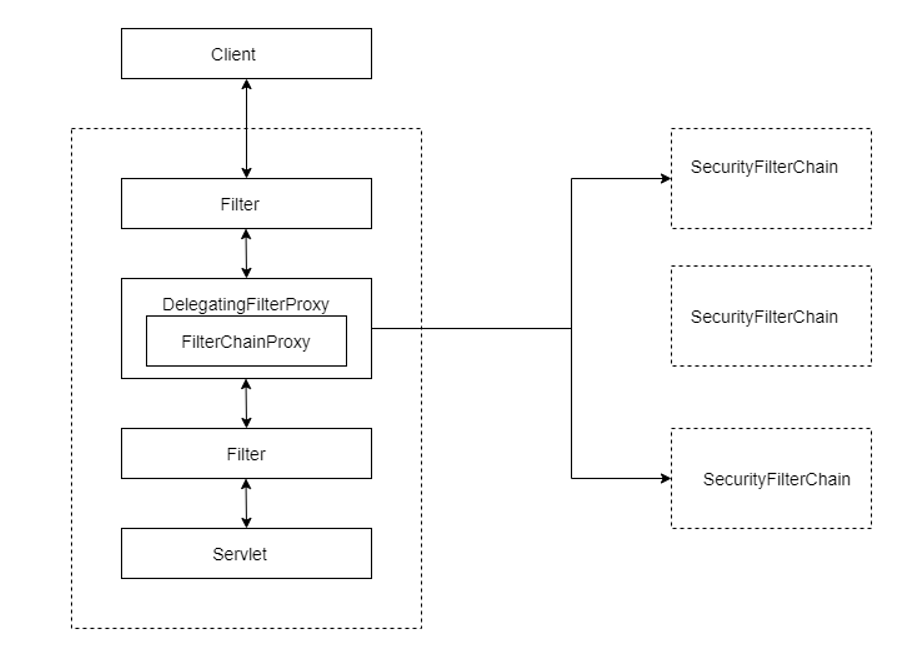
In this section, we’ll discuss high level architecture of Spring Security. Spring Security is based on Servlet Filters. The center of Spring Security is FilterChainProxy
. Lets discuss about it.
FilterChainProxy
is the starting point of Spring Security’s Servlet support.FilterChainProxy
clears out theSecurityContext
which if not done could result in memory leaks.FilterChainProxy
provides control on the condition to invokeSecurityFilterChain
. Generally, filters are invokes based on URL, butFilterChainProxy
allows you to invokeSecurityFilterChain
based onHttpServletRequest
as well.FilterChainProxy
can be used to determine whichSecurityFilterChain
should be used.FilterChainProxy
appliesHttpFireWall
which protects applications from certain attacks like HTTP Response Splitting.
Only the first
SecurityFilterChain
that matches will be invoked. EachSecurityFilterChain
can be unique and can be configured in isolation. It is possible thatSecurityFilterChain
has zero security filters.
5. Security Filters
Security filters are responsible for implementing the security features. There are individual filters which are inserted into the FilterChainProxy
. Following are few security filters:
SecurityContextPersistenceFilter
HeaderWriterFilter
CorsFilter
CsrfFilter
LogoutFilter
OAuth2AuthorizationRequestRedirectFilter
Saml2WebSsoAuthenticationRequestFilter
OAuth2LoginAuthenticationFilter
Saml2WebSsoAuthenticationFilter
UsernamePasswordAuthenticationFilter
OpenIDAuthenticationFilter
DefaultLoginPageGeneratingFilter
DefaultLogoutPageGeneratingFilter
BearerTokenAuthenticationFilter
BasicAuthenticationFilter
RememberMeAuthenticationFilter
As explained in Spring Security official documentation: Spring Security’s Servlet support is contained within FilterChainProxy
. Since FilterChainProxy
is a Bean, it is typically wrapped in a DelegatingFilterProxy
. SecurityFilterChain
is used by FilterChainProxy
to determine which Spring Security Filters should be invoked for this request. The Security Filters in SecurityFilterChain
are typically Beans, and are registered with FilterChainProxy
.
7. Security Exception Handling
ExceptionTranslationFilter
is used to handle security exceptions. ExceptionTranslationFilter
inserted into FilterChainProxy
like other security filters. ExceptionTranslationFilter
provides facility to convert AuthenticationException
and AccessDeniedException
into HTTP responses. If AuthenticationException
or AccessDeniedException
exception is not encountered, then ExceptionTranslationFilter
does nothing.
The process of handling security exception is:
ExceptionTranslationFilter
invokesFilterChain.doFilter(request, response)
method. This is equivalent to invoking the rest of the application.- If user is not authenticated or an
AuthenticationException
, then start authentication. The authentication process is as follows:- The
SecurityContextHolder
is cleared. - The
HttpServletRequest
is saved in theRequestCache
. Once the user is successfully authenticated,HttpServletRequest
saved inRequestCache
is used to continue with the original request. - The
AuthenticationEntryPoint
is used to request credentials from the client.
- The
- If it is an
AccessDeniedException
, thenAccessDeniedHandler
is invoked to handle access denied.
8. Getting Spring Security in Project
8.1 Get Spring Security in Spring Boot project
You can get Spring Security in your project by using spring-boot-starter-security
starter dependency. If you have a Maven project, include the following dependency:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
8.3 Get Spring Security without Spring Boot in Maven project
If you have a Maven project and not using Spring Boot, you can mention like this in your pom.xml
.
<dependencyManagement> <dependencies> <!-- other dependencies to be inserted here --> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-bom</artifactId> <version>{spring-security-version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
Here, spring-security-version
is the version of Spring Security which you want to use. This will download all transitive dependencies as well.
9. Conclusion
In this tutorial, we discussed Spring Security in brief. We discussed architecture of Spring Security. In the upcoming tutorials, we’ll see how Spring Security can be used in application to implement authentication, authorization and prevent common attacks.